Check Email Already Exists with Jquery Ajax
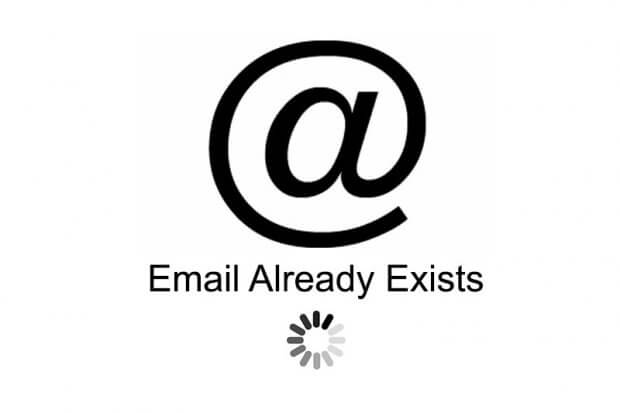
In registration form mostly in web 2.0, email address is required to register for each user. In registration form we need to check email address from the database using Ajax to avoid the email duplicates, and the form tell the user the email inputted is exist and we need to change the email address.
In this this tutorial, we create a simple registration form for the demo and we use jQuery Ajax to request the email to the database, if already exist.
We use Ajax instead of plain PHP, because Ajax can able to talk the database without reloading the browser. We can send the data from the registration form and post the data to the request file. Some sites use Ajax in the registration form to verify the user email or username exist.
See the Demo
Step 1: Create a Database
First create a example database ’email_exists’ you can change if you want, and run the query below, will create ‘users’ table and insert example data.
CREATE TABLE `users` ( `id` int(10) UNSIGNED NOT NULL, `first_name` varchar(50) NOT NULL, `last_name` varchar(50) NOT NULL, `email` varchar(50) NOT NULL ); ALTER TABLE `users`ADD PRIMARY KEY (`id`); ALTER TABLE `users` MODIFY `id` int(10) UNSIGNED NOT NULL AUTO_INCREMENT;
Step 2: Create Database Connection
Create a database connection, input your MySQL host, user, password and database name the ’email_exists’.
database.php
<?php $db = mysqli_connect('localhost', 'root', '', 'email_exists'); if(!$db) { echo mysqli_connect_error(); } ?>
Step 3: Creating the Registration Page
Create a form page, we have a registration form the user can input ‘first name’, ‘last name’ and ’email’ as our example in this tutorial, and also we need the validate the form using jQuery.
index.php
<?php require_once("database.php"); ?> <!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <meta name="robots" content="noindex,nofollow"/> <title>Check Email Already Exists with Jquery Ajax</title> <link href="style/style.css" rel="stylesheet" type="text/css" media="all" /> <script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script> <script type="text/javascript" src="js/script.js"></script> </head> <body> <div id="wrap"> <!--wrap start--> <h1>Check the Email if Already Exist</h1> <form action="" method="post" id="mainform"> <table id="table_data"> <tr> <td>First Name</td> <td><input name="fname" type="text" size="30"></td> <td><span class="fname_val validation"></span></td> </tr> <tr> <td>Last Name</td> <td><input name="lname" type="text" size="30"></td> <td><span class="lname_val validation"></span></td> </tr> <tr> <td>Email</td> <td><input name="email" type="text" size="30"></td> <td><span class="email_val validation"></span></td> </tr> <tr> <td> </td> <td><input name="register" type="button" value="Register"> <span class="loading"></span></td> <td></td> </tr> </table> <div id="note"> <b>Email Already Exist:</b> <span style="color:#B41F2B;">[email protected]</span> </div> </form> </div> <!--wrap end--> </body> </html>
Below the title tag we include the stylesheet the ‘style.css’ and the live jQuery script from google cdn, and lastly the jQuery script.
style/style.css
body { font-family: Arial, Helvetica, sans-serif; font-size:13px; color:#464646 } h1 { font-family: "Helvetica Neue",Helvetica,Arial,sans-serif; margin-left: 25px; } p { margin:10px; padding:10px; color:#000000; } table#table_data { margin-left: 25px; } form#mainform input[type="text"] { border: 1px solid #E5E5E5; margin-bottom: 3px; padding: 5px; } form#mainform input[name="register"] { border: 1px solid #BBBBBB; border-radius: 12px 12px 12px 12px; color: #464646; cursor: pointer; font-size: 13px; margin-top: 10px; padding: 3px 8px; } form#mainform input[name="register"]:hover { border: 1px solid #666666; } div#note { margin-left: 25px; margin-top: 25px; } span.validation { font-style:italic; color:#B41F2B; } span.loading { font-style: italic; left: 5px; position: relative; }
Step 4: Creating the jQuery Ajax Script
js/script.js
jQuery(function($) { var val_holder; $("form input[name='register']").on('click', function() { // form validation val_holder = 0; var fname = jQuery.trim($("form input[name='fname']").val()); // first name field var lname = jQuery.trim($("form input[name='lname']").val()); // last name field var email = jQuery.trim($("form input[name='email']").val()); // email field var email_regex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/; // reg ex email check if(fname == "") { $("span.fname_val").html("This field is empty."); val_holder = 1; } if(lname == "") { $("span.lname_val").html("This field is empty."); val_holder = 1; } if(email == "") { $("span.email_val").html("This field is empty."); val_holder = 1; } if(email != "") { if(!email_regex.test(email)){ // if invalid email $("span.email_val").html("Your email is invalid."); val_holder = 1; } } if(val_holder == 1) { return false; } val_holder = 0; // form validation end // start $("span.loading").html("<img src='images/ajax-loader.gif'>"); $("span.validation").html(""); var datastring = 'fname='+ fname +'&lname='+ lname +'&email='+ email; //var datastring = $('form#mainform').serialize(); // or use serialize $.ajax({ type: "POST", // type url: "check_email.php", // request file the 'check_email.php' data: datastring, // post the data success: function(responseText) { // get the response if(responseText == 1) { // if the response is 1 $("span.email_val").html("<img src='images/invalid.png'> Email are already exist."); $("span.loading").html(""); } else { // else blank response if(responseText == "") { $("span.loading").html("<img src='images/correct.png'> You are registred. redirecting..."); $("span.validation").html(""); $("form input[type='text']").val(''); // optional: empty the field after registration // your redirect code here } } }, timeout: 20000, // timeout if 20 secs error: function (jqXHR, textStatus, errorThrown) { switch(textStatus) { case "timeout": // connection timeout handler alert('Connection Timeout, Please reload the page.'); break; case "error": alert('Connection Error, Please reload the page.'); // connection 404 handler break; default: alert(textStatus); } } }); // ajax end }); // click end });
In the jQuery script we create the simple validation form first for user fails to input the data. In the Ajax there are 4 parameters… type, url, data and return success.
Step 5: The Ajax Request File
We connect to mysql database first then, after sending the data via Ajax, we catch the post data and process to the MySQL query and send back a request and Ajax catch again the request.
check_email.php
<?php require_once("database.php"); // require the db connection /* catch the post data from ajax */ $fname = trim($_POST['fname']); $lname = trim($_POST['lname']); $email = trim($_POST['email']); $query = mysqli_query($db, "SELECT `email` FROM `users` WHERE `email` = '$email'"); if(mysqli_num_rows($query) == 1) { // if return 1, email exist. echo '1'; } else { // else not, insert to the table $query = mysqli_query($db, "INSERT INTO `users` (`first_name` ,`last_name` ,`email`) VALUES ('$fname', '$lname', '$email')"); } ?>
After successfully registered, we will be redirect to this page, the Next page or Home page, you can modify the process registration if you want .
Done
Were done, we creating the check email exist using jQuery Ajax. Let’s have a look at what we’ve achieved:
- We’ve set up a mysql database.
- We’ve create a simple form registration form.
- We’ve write the jQuery Ajax script to able to talk the database without reloading the broswer.
- We’ve write the request file from jQuery Ajax, to get the response of PHP script.
If you enjoyed this article, please consider sharing it!